इस tutorial में हम समझेंगे की C programming में Pointers क्या होता है और सीखेंगे की कैसे आप अपने C programs में pointer declaration, initialization और pointers related operations कर सकते हो.
C programming में Pointers एक ऐसा topic है जिसकी importance आपको तब समझ में आती है जब आप real life में C programming की help से system applications बनाते हैं जैसे की operating system इत्यादि.
ये बात मैंने यहाँ इसलिए बतायी क्योंकि beginner programmers को शुरू में pointers का सिर्फ basic सिखाया जाता है और ये tutorial भी pointers के सभी basic topics को ही cover करेगा.
इसलिए इस tutorial को पढ़कर या इसके examples को देखकर अपने मन में ये राय मत बना लेना की pointers ज्यादा important topic नहीं है या फिर pointers की जरूरत ही क्या है.
C Programming में Pointer क्या है?
जब भी आप C programs में variables, arrays या structures को declare या initialize करते हैं तब उन्हें computer memory (RAM) में space allocate किया जाता है.
हर memory space (location) का एक unique numberic address होता है और अगर हमें इन memory addresses को किसी काम के लिए अपने programs में use करना हो तो इसके लिए हम pointers का use करते हैं.
C programming में pointers special variables होते हैं जो किसी दूसरे variables, arrays या structures के memory location के addresses को points (hold) करते हैं.
मैंने pointers को special variables इसलिए बोला क्योंकि ये normal variables की तरह values hold नहीं करते बल्कि pointer variables memory addresses को points (hold) करते हैं.
Pointer Declaration in C Programming
जैसा की मैंने ऊपर बताया की pointers भी variable ही होते हैं इसलिए pointer variables declaration लगभग normal variable declaration की तरह ही होती हैं.
Pointer variable declaration में बस फर्क ये होता है की pointer variable name से पहले asterisk symbol ( * ) लगाया जाता है और इसी symbol की वजह से compiler को पता चलता है की ये normal variable नहीं बल्कि pointer variable है.
Pointer variable declaration syntax:
data_type *variable_name;
Pointer variable declaration example:
int *ptr, *tmp; char *ct; float *g;
Pointer Initialization in C Programming
Pointer initialization का मतलब होता है की pointer variable को किसी normal variable का address assign करना.
जैसा की आपने ऊपर pointer declaration में देखा की सभी pointer variables के साथ एक data type associated होता है.
जिसका ये मतलब होता है की pointer variable जिस data type का होगा वो सिर्फ उसी data type के variable के address को point (hold) कर सकता है.
जैसे integer pointer सिर्फ integer variable के address को point करेगा और character pointer सिर्फ character variable के address को point करेगा.
अगर आप float variable का address int pointer को assign करेंगे तो compiler आपको कोई error show नहीं करेगा लेकिन शायद आपको आपके program का सही result भी ना मिले.
इसलिए किसी भी data type के variable का address उसी data type के pointer variable में assign करना चाहिए.
Pointer variable को address assign करने के लिए हम reference operator ( & ) का use करते हैं.
Reference operator को हम “Address of Operator” भी कहते है और इस operator के लिए हम जो symbol ( & ) use करते हैं उसे ampersand कहते हैं.
किसी भी variable, array या structure के नाम के आगे reference operator ( &) use करने से आप उसका memory address get कर सकते हो.
Pointer variable initialization syntax:
data_type *variable = &variable; pointer_variable = &variable; pointer_variable = &array_name; pointer_variable = &structure_name;
अगर आप pointer variable declaration के बाद pointer variable को address assign करते हैं तो तब आपको pointer variable के साथ asterisk ( * ) नहीं लगाना होता है.
जैसा की आप ऊपर syntax में first pointer initialization के अलावा बाकी के तीनों pointer initializations में देख सकते हो.
Pointer variable initialization example program:
#include <stdio.h>
int main()
{
int num, *ptr;
num=25;
ptr = #
printf("Value of Num : %d\n",num);
printf("Address of Num : %d\n",&num);
printf("Value of Ptr : %d\n",ptr);
printf("Address of Ptr : %d\n",&ptr);
return 0;
}
Output:
Value of Num : 25 Address of Num : 6356728 Value of Ptr : 6356728 Address of Ptr : 6356432
Explanation:
जैसा की ऊपर example में देख रहे हैं की हमने int data type का एक normal variable और एक pointer variable declare किया है.
दोनों variables declare होते ही दोनों variables को memory में space (location) allocate कर दिया जाएगा और जैसा की मैंने ऊपर बताया था की सभी memory locations का unique address होता है.
Variables declare करने के बाद हमने num variable को value 25 assign कर दी और pointer variable ptr को variable num का address assign कर दिया यानी num की value 25 है और ptr की value num variable का address.
इसलिए जब आप ptr variable की value print कराएँगे तब आपको output में variable num का address मिलेगा.
आप variable num से भी उसका address print करा सकते हैं बस आपको variable print करते वक्त उसके साथ Reference operator (&) का use करना पड़ेगा जैसे हमने ऊपर example में किया है.
Note: क्योंकि memory location address numeric form में होता है इसलिए उसे print कराने के लिए हम %d format specifier का use करते हैं.
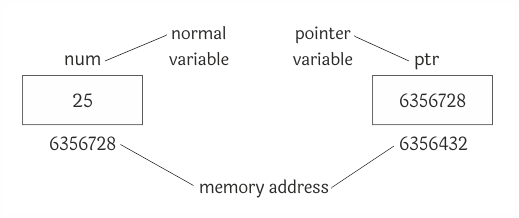
Dereference Operator ( * ) in C Programming
जब आप variable declaration के वक्त asterisk ( * ) उस variable से पहले लगाते हैं तो उसका मतलब होता है की आप उस variable को pointer (variable) बनाना चाहते हो.
लेकिन जब आप pointer declare करने के बाद pointer variable के साथ asterisk ( * ) का use करते हो तब उस asterisk को हम “dereference operator” या “value at operator” या “indirection operator” कहते हैं.
इसलिए जब आप pointer declare करने के बाद उस pointer को अपने program में use करते वक्त उसके साथ dereference operator ( * ) लगाते हैं तब उस process को हम pointer dereferencing कहते हैं.
Pointer dereferencing का ये मतलब है की आप किसी pointer variable की help उस value को get करना चाहते हो जो उस address पर मौजूद है जिस address को वो pointer point कर रहा है.
यानी आप pointer की help से किसी variable के address को भी access कर सकते हो और pointer के साथ dereference operator का use करके आप variable की value को भी access कर सकते हो.
Pointer Dereferencing Example Program:
#include <stdio.h>
int main()
{
int num, *ptr;
num=20;
ptr = #
printf("Using Num Variable\n");
printf("Value of Num : %d\n",num);
printf("Address of Num : %d\n",&num);
printf("\n\nUsing Pointer \n");
printf("Value of Num : %d\n",*ptr);
printf("Address of Num : %d\n",ptr);
return 0;
}
Output:
Using Num Variable Value of Num : 20 Address of Num : 6356732 Using Pointer Value of Num : 20 Address of Num : 6356732
Explanation:
Pointer ptr के पास variable num का address है इसलिए जब आप ptr की value print कराएँगे तब वास्तव में variable num का address ही print होगा और जब आप pointer के साथ dereference operator ( * ) का use करेंगे बी variable num की value print होगी.
Void Pointer in C Programming
जैसा की हमने ऊपर पढ़ा की किसी भी data type के address को store करने के लिए उसी data type का pointer होना चाहिए.
लेकिन कभी-कभी हमें अपने program में ऐसा pointer चाहिए होता है जो किसी भी data type के address को store (point) कर सकें तो इसके लिए हम void pointer का use करते हैं.
void pointer syntax:
void *pointer_name;
जैसा की आप ऊपर syntax में देख रहे हो की void pointer बनाने के लिए हमने void data type का use किया है.
void pointer को हम typeless pointer और generic pointer भी कहते हैं और इसलिए void pointer किसी भी data type के address को store कर सकता है.
void pointer की सबसे अच्छी बात ये होती है की आप void pointer को बिना explicit type casting के किसी दूसरे data type के pointer में cast (convert) कर सकते हो.
void pointer example program:
#include <stdio.h>
int main()
{
void *ptr;
int num;
char ch;
ptr = #
printf("Address of num : %d\n",&num);
printf("Address of num : %d\n",ptr);
ptr = &ch;
printf("Address of ch : %d\n",&ch);
printf("Address of ch : %d\n",ptr);
return 0;
}
Output:
Address of num : 6356728 Address of num : 6356728 Address of ch : 6356727 Address of ch : 6356727
Explanation:
ऊपर program में हमने ptr नाम का void pointer declare किया है और पहले उसमें int data type के variable का address store करके print कराया है और फिर उसके बाद char data type के variable का address store करके print कराया है.
अगर हमारा ptr pointer int data type का होता तो वो सिर्फ variable num का address ही store कर सकता था यानी आप उसमें variable ch का address store नहीं करा सकते थे.
Dereferencing a void pointer in C
जैसा की मैंने आपको ऊपर बताया था की pointer dereferencing का मतलब होता है की pointer की help उस address की value को निकलना जिस address को pointer store किया हुआ है.
लेकिन आप void pointer से directly dereferencing नहीं कर सकते हो. इसके लिए आपको पहले void pointer को उस data type के pointer में cast करना होगा जिस data type के address को वो store किया हुआ है.
void pointer type casting syntax:
data_type *pointer_name = (data_type *) void_pointer;
void pointer type casting and dereferencing example program:
#include <stdio.h>
int main()
{
void *vptr;
int num,*iptr;
num = 20;
vptr = #
iptr = (int *)vptr;
printf("Address of num : %d\n",iptr);
printf("Address of num : %d\n",vptr);
printf("Value of num : %d\n",*iptr);
printf("Value of num : %d",*(int *)vptr);
return 0;
}
Output:
Address of num : 6356724 Address of num : 6356724 Value of num : 20 Value of num : 20
Explanation:
ऊपर program में हमने सबसे पहले line 9 पर int variable num का address void pointer vptr को assign किया है.
इसके बाद line 11 पर हमने void pointer को type cast करके int pointer में assign कर दिया है यानी अब int pointer iptr भी उसी address को point कर रहा होगा जिसे void pointer vptr कर रहा है.
इसलिए जब हमने line 13 और 14 पर vptr और iptr की value को print करवाया है तब variable num का address print हुआ है.
line 16 पर हमने int pointer iptr को dereference करके variable num की value को print करवाया है.
line 17 पर क्योंकि void pointer से हम dereferencing कर रहें जो की directly हो नहीं सकती इसलिए हमने पहले void pointer को int pointer में cast किया है फिर उसके बाद pointer derefernce किया है.
What’s Next: इस tutorial में हमने C Pointers के बारे में पढ़ा. Next tutorial में हम C programming में Pointer Arithmetic का use करना सीखेंगे