C programming language के इस हिंदी tutorial में हम ये सीखेंगे की call by value and call by reference में क्या difference और इनका क्या use है.
Call by value and Call by reference को अच्छी तरह से समझने के लिए आपको पहले C functions और C pointers की पूरी जानकारी होना जरूरी है इसलिए पहले इन tutorials को जरूर पढ़ लें.
जैसा की आपने C functions वाले tutorial में पढ़ा था की जब हम किसी parameterized functions को call करते हैं तब हम arguments pass करते हैं.
Function arguments को आप दो तरह से pass कर सकते हो पहला value के तौर पर दूसरा reference के तौर पर. अब इन दोनों में क्या अंतर है ये हम एक-एक करके समझेंगे.
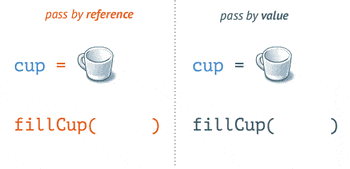
Function Call By Value
Functions वाले tutorial में आपने जितने भी parameterized function वाले example programs देखे वो सभी call by value के ही examples थे.
जब आप function call करते वक्त variables को argument के तौर पर pass करते हो तब होता ये है की उन variables के pass जो values होती है वो pass होती है ना की वो variables.
आप इसे ऐसे भी समझ सकते हो की function arguments की values function parameters में copy (assign) हो जाती हैं.
इसलिए जब function के अंदर अगर parameters की values में कोई interchange होता है तो उसका कोई भी effect arguments पर नहीं पड़ता है.
Call by value को function के साथ समझने से पहले simple variables के साथ समझते हैं और फिर function के साथ समझेंगे.
#include<stdio.h>
int main()
{
int a,b;
a=10;
b=a;
printf("Value of A : %d\n",a);
printf("Value of B : %d\n",b);
b=20;
printf("Value of A : %d\n",a);
printf("Value of B : %d\n",b);
return 0;
}
Output:
Value of A : 10 Value of B : 10 Value of A : 10 Value of B : 20
Explanation:
ऊपर program में जब हमने line 7 पर variable a को variable b में assign किया तब actual में हुआ ये होगा की variable a की value variable b को assign (copy) हो गयी होगी.
अब आपको ये समझना है की variable a ने सिर्फ अपनी value variable b को assign की है उनमें किसी तरह का कोई relation (link) connect नहीं हुआ है.
इसलिए जब line 12 पर हमने variable b की value को update (change) किया है तो इसका किसी तरह का कोई भी effect variable a पर नहीं पड़ेगा.
Swap two numbers using call by value:
#include<stdio.h>
void swap(int x,int y);
int main()
{
int a,b;
a=5;
b=7;
printf("Before Function Call:-\n");
printf("Value of A : %d\n",a);
printf("Value of B : %d\n",b);
swap(a,b);
printf("After Function Call:-\n");
printf("Value of A : %d\n",a);
printf("Value of B : %d\n",b);
return 0;
}
void swap(int x,int y)
{
int z;
z=x;
x=y;
y=z;
}
Output:
Before Function Call:- Value of A : 5 Value of B : 7 After Function Call:- Value of A : 5 Value of B : 7
Explanation:
ऊपर program में line 14 पर जब हमने swap() function को call किया है तब हमने variable a और b की value को argument के तौर पर pass किया है.
इसलिए variable a की value swap() function के parameter (variable) x में copy हो जाएगी और इसी तरह variable b की value swap() function के parameter y में copy हो जाएगी.
उसके बाद हमने function के अंदर variables x और y की value को swap (interchange) किया है लेकिन इस swapping का असर main() function के variables a और b पर नहीं पड़ेगा.
Function Call by Reference
Call by reference ये होता है की जब आप function call करते हैं तब आप arguments के तौर पर variables की value की बजाय उनका address (reference) pass करते हैं.
इसलिए function parameters में normal variables की बजाय pointer variables का use होता है जिससे की वो arguments में pass किये गए address को store (point) कर सकें.
उसके बाद अगर आप उन pointers की help से function के अंदर कोई changes करते हो उसका effect उन variables में होगा जिनका address आपने arguments के तौर पर pass किया था.
Call by reference को function के साथ समझने से पहले simple variables के साथ समझते हैं और फिर function के साथ समझेंगे.
#include<stdio.h>
int main()
{
int a,*b;
a=10;
b=&a;
printf("Before: Value of A : %d\n",a);
*b=20;
printf("After: Value of A : %d\n",a);
return 0;
}
Output:
Before: Value of A : 10 After: Value of A : 20
Explanation:
ऊपर program में हमने line 7 पर variable a के address को pointer b में assign किया इसलिए pointer b अब variable a के address को point (store) करेगा.
इसलिए अब एक तरह से इन दोनों में एक तरह का relation (link) बन गया और इसलिए जब हमने line 12 पर pointer b के साथ dereference operator ( * ) का use करके pointer b को value assign की है तब actual में वो value variable a को assign हुई होगी.
क्योंकि जब आप किसी pointer के साथ dereference operator का use करके उसे कोई value assign करते हो तो वो value उस variable में store होती है जिस variable के address को pointer ने store किया हुआ है.
Swap two numbers using call by reference:
#include<stdio.h>
void swap(int *x,int *y);
int main()
{
int a,b;
a=5;
b=7;
printf("Before Function Call:-\n");
printf("Value of A : %d\n",a);
printf("Value of B : %d\n",b);
swap(&a,&b);
printf("After Function Call:-\n");
printf("Value of A : %d\n",a);
printf("Value of B : %d\n",b);
return 0;
}
void swap(int *x,int *y)
{
int z;
z=*x;
*x=*y;
*y=z;
}
Output:
Before Function Call:- Value of A : 5 Value of B : 7 After Function Call:- Value of A : 7 Value of B : 5
Explanation:
ऊपर program में line 14 पर जब हमने swap() function को call किया है तब हमने variable a और b के address को argument के तौर पर pass किया है.
इसलिए variable a का address swap() function के parameter (pointer) x में copy हो जाएगा और इसी तरह variable b का address swap() function के parameter y में copy हो जाएगा.
उसके बाद हमने pointer x और y के साथ dereference operator ( * ) का use करके variable a और b की value को swap (interchange) किया है.
इसलिए swap() function call के बाद जब हमने दोबारा से variable a और b की value को print कराया तो वो interchanged होकर print हुई.