इस tutorial में हम सीखेंगे की कैसे आप C programs में CodeBlocks और Command Prompt की help से command line arguments input ले सकते हो.
जैसा की हमने C functions वाले tutorial में पढ़ा था की function या तो parameterized होगा या without parameterized होगा.
अगर function parameterized है तो इसका मतलब है की जब आप उस function को call करेंगे तब आप कुछ values pass करेंगे जिन्हें हम arguments कहते हैं.
इसी तरह हमारे program में जो main() function होता है वो भी parameterized या without parameterized हो सकता है.
लेकिन अब क्योंकि आप खुद से main() function को call नहीं करते हो इसलिए आप अन्य functions की तरह main function को arguments pass नहीं कर सकते हो.
Parameterized main() function को arguments pass करने के लिए हमें अपने program को command line से run करना होता है.
इसलिए C program को command line से run करते वक्त main function को हम जो arguments pass करते हो उन्हें हम Command Line Arguments कहते हैं.
Command Line Arguments क्या है?
अभी तक आपने सभी C programs में main function को without parameters ही define किया होगा जैसा की आप नीचे syntax में देख सकते हो.
int main() { ............ ............ }
Command line arguments pass (input) करने के लिए आपको main function में two predefined parameters define करने होते हैं जैसा की आप नीचे syntax में देख सकते हो.
int main(int argc, char *argv[]) { ............ ............ }
argc (Argument Count): ये parameter एक integer variable होता है और इस variable में ये store होता है की array argv में कितनी values store हैं. आप ऐसा भी कह सकते हैं की ये variable array argv की length को store करता है.
argv (Argument Values): ये parameter एक string data type का array होता है और इसमें वो सभी arguments (values) store होते हैं जो command line से input (pass) किये जाते हैं.
अब क्योंकि ये string array है इसलिए सभी arguments के तौर पर input की गयी सभी values इस array के अलग-अलग index पर string की form में store हो जाती है.
इस array के 0th index पर अपने आप हमारे C program का name store होता है और उसके बाद के सभी indexes पर वो सभी command line arguments जो हम input करेंगे.
इस tutorial में पहले हम Command Prompt पर command line arguments input लेना सीखेंगे और उसके बाद Codeblocks में.
अगर मैं अपनी निजी राय दूँ तो command line arguments input करने के लिए मैं पहला तरीका यानी program को command prompt से run करके command line arguments input करना ज्यादा पसंद करता हूँ.
Command Line Arguments Input कैसे लें?
C programs में command line arguments input लेने का पुराना तरीका तो ये ही है की आप अपने C program को command line पर run करें.
लेकिन अब क्योंकि ज्यादातर programmers अपने C programs को command line की बजाय CodeBlocks, Turbo C++ जैसे IDEs पर run करते हैं इसलिए हम दोनों तरीकों से command line arguments input लेना सीखेंगे.
Command line पर arguments input (pass) करने के लिए सबसे पहले आपको अपने computer में C compiler install और setup करना होगा.
उसके बाद आपको सीखना होगा की कैसे आप अपने C programs को command line पर compile and run कर सकते हो.
ये सब सीखने के लिए आपको कहीं जाना नहीं है बस आपको नीचे दिए गए link पर click करके हमारा tutorial पढ़ना है और फिर उसके बाद इस tutorial को आगे पढ़ना है.
Read: Compile and Run C Programs in Command Line using GCC
अब क्योंकि आपने C programs को command line पर compile और run करना सीख लिया है इसलिए अब हम सीखेंगे की कैसे आप command line पर arguments input कर सकते हो.
Command Line Arguments Syntax:
program.exe argument1 argument2 agrument3 ... agrumentN
Syntax Explanation:
हम command line arguments तब input करते हैं जब हम अपने program को run करते हैं और हम अपने सभी arguments C program की executable file के बाद input करते हैं.
Command Line Arguments Example Program 1:
#include<stdio.h>
int main(int argc,char *argv[])
{
printf("Value of Argc : %d",argc);
printf("\n0th Index : %s",argv[0]);
return 0;
}
Compile and Run:
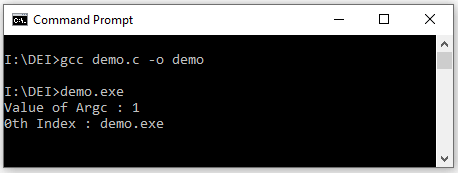
Program Output:
Value of Argc : 1 0th Index : demo.exe
Output Explanation:
जैसा की आप ऊपर देख सकते हो की जब हमने अपने C program को run किया है तब हमने कोई भी arguments input नहीं किया है.
लेकिन जब हम variable argc print कराया तब 1 print क्योंकि जैसा की मैंने बताया की array argv के 0th index पर अपने आप program name insert (input) हो जाता है.
Command Line Arguments Example Program 2:
#include<stdio.h>
int main(int argc,char *argv[])
{
int i;
for(i=0;i<argc;i++)
{
printf("\n%d index value : %s",i,argv[i]);
}
printf("\n\n");
return 0;
}
Compile and Run:
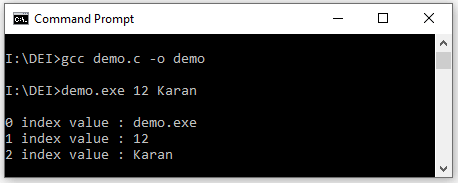
Program Output:
0 index value : demo.exe 1 index value : 12 2 index value : Karan
Output Explanation:
ऊपर जब हमने program को run किया तब हमने 2 arguments को भी साथ में input किया है यानी program name के साथ-साथ ये दोनों arguments भी parameter argv (array) में store हो जाएंगे और variable argc में अपने आप number 3 store हो जाएगा.
इसके बाद हमने variable i और argc की help से loop चला कर array argv के सभी elements (values) को print करा दिया है.
CodeBlocks में Command Line Arguments Input कैसे लें?
CodeBlocks में command line arguments को program arguments कहा जाता है और program arguments set (input) करने के लिए आपको सबसे पहले project create करना पड़ेगा.
CodeBlocks में project create करने के लिए आपको File Menu >> New >> Project पर click करना होगा.
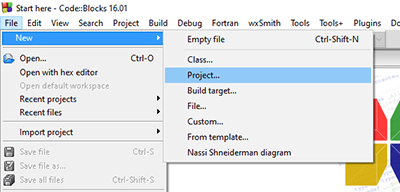
इसके बाद आपको सामने project category select करने के लिए dialog box आएगा. उसपर आपको Empty project पर click करके Go और फिर Next पर click करना है.
अब आपके सामने नीचे दिया गया dialog box open हो जाएगा जिसमें आपको सबसे पहले project title type करना है जो आप कुछ भी enter कर सकते हो.
उसके बाद आप वो location select कर लीजिये जहाँ पर आप अपने project का folder create करना चाहते हो.
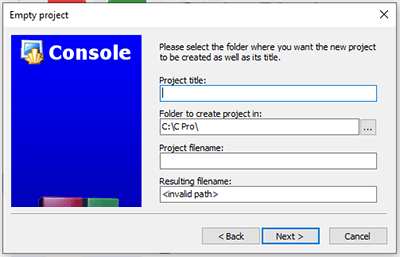
इसके बाद next पर click करके finish button पर click कर दीजिएगा. अब आपको File Menu >> New >> Empty File पर click करना है.
अब अगर आपके सामने कोई dailog box show हो तो उसे yes button पर click कर दीजिएगा और फिर अपनी C program file किसी भी name से save कर दीजिएगा लेकिन file name के बाद .c extension जरूर लगा दीजिएगा.
अब आप अपना कोई भी C program बना लीजिए जिसमें आप command line arguments input लेना चाहते हो . मैं आपको नीचे दिए गए program को run करके दिखाता हूँ.
Command Line Arguments Example Program 3:
#include<stdio.h>
int main(int argc,char *argv[])
{
int i;
for(i=0;i<argc;i++)
{
printf("\n%d index value : %s",i,argv[i]);
}
printf("\n\n");
return 0;
}
Set Command Line Arguments in Codeblocks:
Codeblocks में command line arguments set करने के लिए आपको Project menu पर click करके Set program arguments पर click करना होगा.
इसके बाद program arguments field में अपने arguments input कर दीजिए जैसे मैंने तीन names (Karan, Rohit, Sanjay) input किया है.
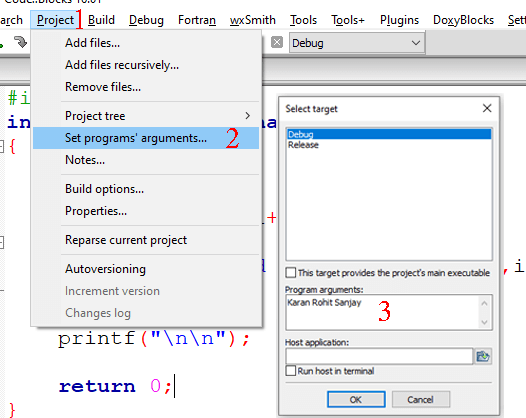
Output:
0 index value : C:\C Pro\Dummy\bin\Debug\Dummy.exe 1 index value : Karan 2 index value : Rohit 3 index value : Sanjay
इस tutorial में हमने सिर्फ ये की आप command prompt और codeblocks में command line arguments input कैसे ले सकते हो. इस topic के practical example programs के लिए आप नीचे दिए link पर click करके उन्हें समझ सकते हो.