Python इस tutorial में हम बात करेंगे Class and Object के बारे में और कैसे आप Python में Classes और Objects बना सकते हो और साथ-साथ self parameter और __init__() method के बारे में भी बात करेंगे.
जैसा की आपको पता है की Python एक बहुत ही popular programming language है जिसमें आप procedural और object-oriented दोनों तरह से programming कर सकते हो.
अगर आपको simple और small projects पर काम करना है तो आप procedural programming कर सकते हैं लेकिन जब आपको बड़े और complex projects पर काम करना होता है तब आपको code बहुत ही organize way में करना होता है और इसके लिए सबसे अच्छा होता है object oriented programming करना.
Object-oriented programming (OOP) में बहुत सारे concept होते हैं और उन सबमें सबसे पहले आपको class और object के बारे में समझना होता है इसलिए आइये समझते हैं की class और object क्या होता है.
What is a Class and Objects in Python?
जब कोई builder किसी colony में एक से ज्यादा घर बनाना चाहता है तब वो पहले एक घर का blueprint (नक्शा) तैयार कराता है और फिर उसी एक blueprint से वो builder कितने भी घर को बनवा सकता है.
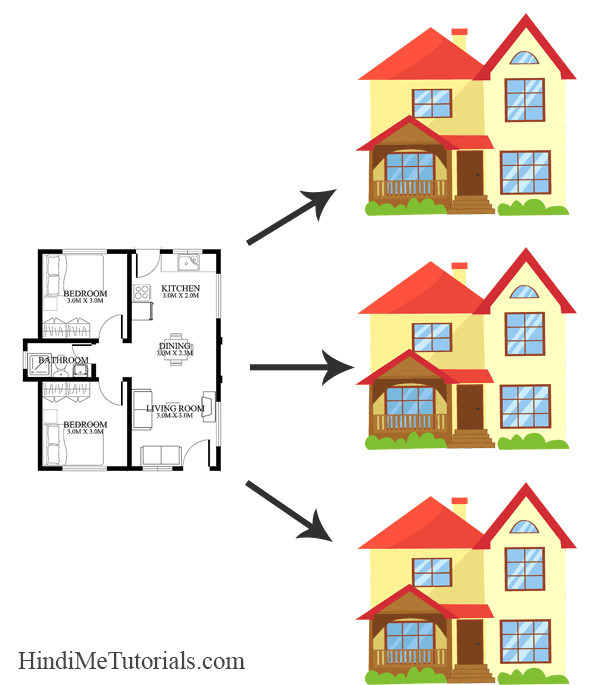
ठीक ऐसे ही जब कोई company किसी new product को manufacture करती हैं तो वो सबसे पहले उस product की एक template (blueprint / sample) तैयार करती है जिसमें उस product की सभी properties और functions define होते हैं और फिर उसी एक template के जरिये वो कंपनी बहुत सारे products बनाती हैं.
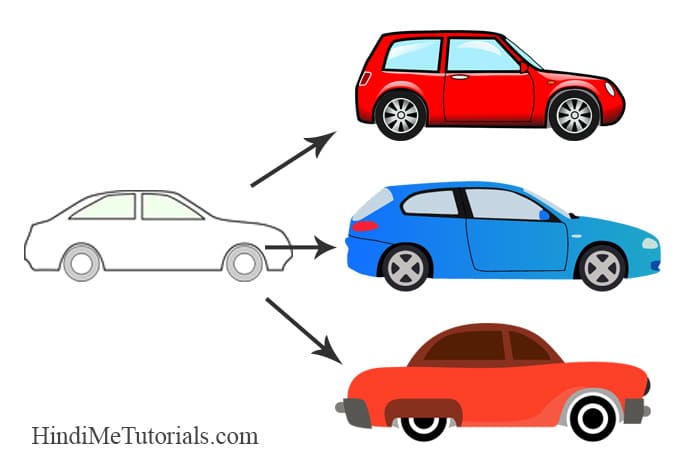
किसी भी entity (चीज) की template एक logical entity होती है जिसमे उस entity की सभी properties और functions को logically group किया जाता है और ये template real world में कोई space acquire नहीं करती है.
Template (blueprint) की हेल्प से जो real world objects create किये जाते हैं वो सब physical entities होते हैं यानी उनके अन्दर मौजूद properties और functions को हम use कर सकते हैं और ये सब objects real world में space भी acquire करते हैं.
किसी एक template से बने हर एक objects के पास template में मौजूद हर एक properties और functions की अपनी अलग copy होती है, जैसे Car template से बनी हर real car में वो सभी properties और functions होंगे जो Car template में logically group किए गये थे.
इसी तरह object-oriented programming में class के अंदर related variables और methods को logically group करके class को template के तौर पर use करते हैं और फिर class से objects बनाकर variables और methods को use करते हैं.
जैसे मान लीजिये हम Car से related कोई Python program बना रहे हो जिसमें हमें Car की multiple properties को store करना है जैसे की Car brand, model, color, speed, price इत्यादि. अब इन सब properties को store करने के लिए हमें अलग-अलग data type के multiple variables declare करने होंगे.
इसी तरह Car के different functions (काम) को define करने के लिए हमें अलग-अलग methods (functions) बनाने होंगे जैसे की start(), moveForward(), moveBackward(), stop(), turnLeft(), turnRight(), honk() इत्यादि.
अब क्योंकि ये सभी variables और methods Car से related (संबंधित) हैं इसलिए हमें कुछ ऐसा करना चाहिए ये सब किसी तरह एक single entity में group हो सकें और फिर उस single entity को हम template एक तौर पर use कर सकें और इस काम के लिए हम Python में class concept को use करते हैं.सभी properties (variables) और functions (methods) को Class की हेल्प से एक template (blueprint) के तौर पर group कर देने के बाद हम इस class के कितने भी Objects बना सकते हैं.
Syntax Example:
#Car class with multiple variables and methods
class Car:
def __init__(self, brand, model, color, speed, price):
self.brand = brand
self.model = model
self.color = color
self.speed = speed self.price = price
def start(self):
#statements
#statements
def moveForward(self):
#statements
#statements
def moveBackward(self):
#statements
#statements
def turnLeft(self):
#statements
#statements
def turnRight(self):
#statements
#statements
def honk(self):
#statements
#statements
def stop(self):
#statements
#statements
#create objects of car class
firstCar = new Car('BMW','X5','Blue',400,9800000)
secondCar = new Car('Kia','Seltos','White',300,1100000)
Difference Between Class and Object in Hindi
- Class एक template (blueprint) होती है और Class के instance को हम Object कहते हैं.
- Class एक logical entity होती है और Object एक physical entity होती है.
- Class को memory में space allocate नहीं किया जाता है लेकिन Object को memory में space allocate किया जाता है.
- Class को एक बार create किया जाता है और लेकिन उस class से आप कितने भी Objects बना सकते हो.
Difference Between Method and Function in Python
Python के इस tutorial तक पहुँचने से पहले ही Python में functions क्या होता है और हम उन्हें कैसे use करते हैं ये तो आप पढ़ (सीख) ही चुकें होंगे और अभी तक आपने Python में functions का use नहीं सीखा है तो पहले Python functions वाला tutorial पढ़ें उसके बाद इस tutorial को आगे continue करें.
जब कोई student Java या C सीखकर Python सीखना शुरू करता है तो उसे function और methods को लेकर बड़ा ही confusion होता है क्योंकि C programming में functions होते हैं और Java में methods लेकिन Python में ये दोनों होते हैं.
वैसे तो functions और methods लगभग एक जैसे ही होते हैं और दोनों के जरिए ही हम किसी specific task को perform करते हैं लेकिन फिर भी दोनों में थोड़ा सा फर्क होता है और इसी फर्क को अब हम समझने वाले हैं.
Functions को किसी भी class के बाहर बनाया जाता है और ये पूरी तरह से independent होते हैं यानी इन्हें call करने के लिए हमें किसी class और object की जरुरत नहीं होती है.
वो functions जिन्हें हम किसी class के अन्दर define करते हैं उन्हें हम methods कहते हैं और ये methods class और उसके object पर dependent होते हैं यानी इन्हें call करने के लिए हमें किसी class और object की जरुरत होती है.
Functions में self name से कोई extra parameter नहीं होता है लेकिन Methods में extra first parameter होता ही है जिसका name ज्यादातर self ही रखा जाता है. आइए अब self parameter के बारे में थोड़ा detail में समझते हैं.
Self Parameter in Python
Python class में हम जो भी methods defined करते हैं उन सभी में first parameter extra होता है और method को call करते वक्त इस first parameter की value हम pass नहीं करते है बल्कि Python खुद से internally इस first parameter की value pass करती है.
अगर class के अन्दर हमें without parameters के method बनाना है तब भी ये एक extra parameter होता ही है और इस method को call करते वक्त हम कोई भी argument pass नहीं करते हैं.
वैसे तो आप इस extra first parameter को कोई भी name दे सकते हैं लेकिन अगर आप common practice की बात करें तो इसका name self ही रखा जाता है.
ये self name का extra first parameter class के current instance (object) को represents करता है. आप इसे बिल्कुल C++ के this pointer और Java programming के this keyword जैसा मान सकते हो.
जब आप किसी Python class के object के किसी method को call करते हैं तब उस method का ये self parameter उस current object को represent करता है जिस object ने इस method को call किया है.
इसके बाद method के अन्दर self parameter की हेल्प से हम current object के variables और methods को access करते हैं.
Syntax:
class Student:
def setData(self, arguments)
#method definition
def displayInfo(self):
#method definition
__init__() Method in Python
Python में कुछ खास methods होते हैं जिन्हें हम magic methods कहते हैं. ये सभी methods special होते हैं क्योंकि ये सभी किसी specific process पर internally अपने आप call होते हैं. Magic methods के name के आगे और पीछे double underscores (__) होते हैं जैसे की __add__(), __len__(), __str__().
__init__() भी एक magic method है और जब भी आप किसी class का object बनाते हैं तब उस class में मौजूद __init__() method अपने आप call होता है. इस method की हेल्प से हम उस object के attributes (variables) को initialize करते हैं.
जैसे C++ और Java में constructor की हेल्प से आप object के instance variables को initialize करते हो ठीक इसी तरह Python में init method की हेल्प से object के instance variables को initialize करते हैं.
अगर आप class में init method without parameter बनाते हैं तो class का object बनाते वक्त आप कोई argument pass नहीं करेंगे और अगर आपका init method with parameters है तो class का object बनाते वक्त आप parameters के according argument pass करेंगे जैसा की method calling के वक्त करते हैं.
Syntax:
class ClassName:
def __init__(self, arguments):
# method definition
How to create Class and Objects in Python?
Python में function को define करने के लिए जैसे हम def keyword का use करते हैं ठीख ऐसे ही Python में class को define करने के लिए class keyword का use होता है.
Syntax:
class ClassName: # class definition # class definition
Class Name: जैसे आप variables और functions को अपने मन से कोई भी name assign करते हो ठीक ऐसे ही आप class को भी अपने मन से कोई भी meaningful name दे सकते हो.
Class name को हम camel case में लिखते हैं यानी हर word का first letter capital लिखा जाता है. ऐसा करना syntax का part नहीं होता है लेकिन ये एक good programming practice होती है.
Examples: Students, MyData, CreateAccount
Class Definition: इसमें हम class attributes (variables) को declare करते हैं और साथ ही उसके methods को define करते हैं.
Class define करने के बाद आप class के कितने भी objects बना सकते हो और इसके लिए आपको बस class को function की तरह call करना होता है.
Syntax:
object_name = ClassName()
Object Name: जैसे integer या string values को store करने variables का use करते हैं ऐसे ही किसी भी class के objects को store (refer) करने के लिए आपको एक reference variable (object name) की जरुरत होती है.
इसी object name (reference variable) की हेल्प से हम object में मौजूद variables और methods को call करते हैं.
ClassName(): इसके जरिए आप class का object create करते हैं और साथ ही साथ object के __ini__() method को call करते हैं. अगर init method parameters के साथ है तो arguments pass करेंगे अन्यथा नहीं.
आइये अब एक complete program बनाकर Python में class और object को अच्छी तरह से समझते हैं.
Example Program:
class Employee:
def __init__(self, name, des, salary):
self.name = name
self.des = des
self.salary = salary
def displayEmp(self):
print("Name of Employee is: ", self.name)
print("Designation of Employee: ", self.des)
print("Salary of Employee: ", self.salary)
e1 = Employee("Karan", "Manager", 50000)
e2 = Employee("Aman", "Developer", 35000)
e3 = Employee("Rohit", "Designer", 30000)
e1.displayEmp()
e2.displayEmp()
e3.displayEmp()
Program illustration:
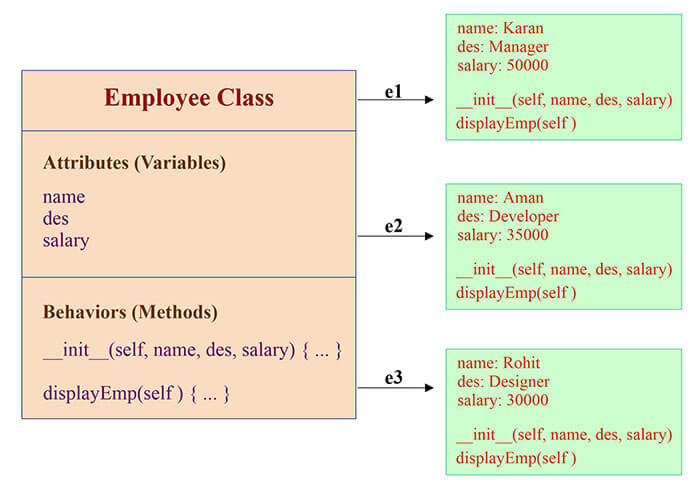
Program Output
Name of Employee is: Karan Designation of Employee: Manager Salary of Employee: 50000 Name of Employee is: Aman Designation of Employee: Developer Salary of Employee: 35000 Name of Employee is: Rohit Designation of Employee: Designer Salary of Employee: 30000
Program Explanation:
ऊपर program में हमने Employee name से एक class बनायीं है और फिर line no. 13, 14, 15 पर हमने Employee class के तीन objects बनाए हैं और जैसा की मैंने आपको ऊपर बताया था किसी class के हर objects के पास class में मौजूद सभी variables और methods की अपनी copy होती है यानी ऊपर तीनो objects के पास अपना अलग-अलग init और displayEmp method होगा.
आप ऊपर class में देख सकते हो की __init__() method में 4 parameter है और जैसा की बताया था की first parameter self name से होता है जिसकी हेल्प से हमने init method के अन्दर object के attributes (variables) को बाकी के 3 parameters की values को assign किया है.
इसके बाद लाइन 17, 18, 19 पर हमने उन तीनो objects के displayEmp method को call किया है और इस method में हमने objects के attributes (variables) को print कराया है.