इस tutorial में, हम Python के 3 important jumping control statements — break, continue, और pass के बारे में चर्चा करेंगे। साथ ही, practical examples की मदद से इन तीनों का उपयोग समझेंगे।
Break Statement in Python
जब तक loop पूरा नहीं होता, तब तक उसके अंदर के statements लगातार execute होते रहते हैं। लेकिन, कभी-कभी हम किसी specific condition के true होने पर loop से बाहर निकलना चाहते है तब हम loop के अंदर break statement का उपयोग करते हैं।
जैसे ही for या while loop के अंदर break statement मिलता है, प्रोग्राम का flow तुरंत loop के बाहर, यानी loop के ठीक बाद मौजूद statements पर चला जाता है।
Note: आम तौर पर, break statement का उपयोग loop में मौजूद decision-making statements, जैसे कि if…else, के अंदर किया जाता है।
Break Statement Syntax:
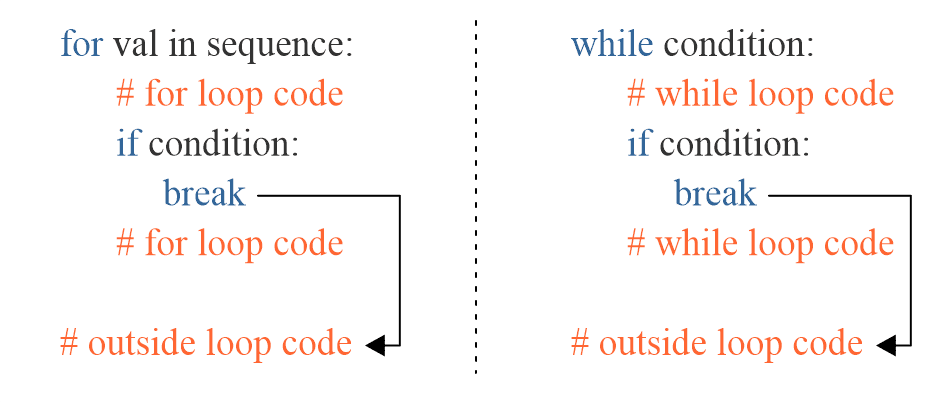
Break Statement with For Loop:
जैसा कि हम अपने for loop वाले tutorial में पढ़ चुके हैं, Python में for loop का उपयोग ज्यादातर किसी sequence (जैसे कि list, tuple, string या range) पर iterate करने के लिए किया जाता है।
for loop के अंदर break statement का उपयोग करके, आप sequence के सभी items पर iterate करने से पहले किसी specified condition के आधार पर loop से exit कर सकते हैं।
Example: नीचें दिए गए example में, हम for loop की मदद से list पर iterate कर रहे हैं। यहां, हमारा उद्देश्य यह है कि जैसे ही list का कोई element number 5 से divisible हो, for loop रुक जाए और प्रोग्राम का flow for loop से बाहर आ जाए।
mylist = [24, 16, 28, 45, 78, 98]
for ele in mylist:
if ele % 5 == 0:
break
print('Element:', ele)
Output:
Element: 24
Element: 16
Element: 28
Break Statement with While Loop:
जैसा कि हम अपने while loop वाले tutorial में पढ़ चुके हैं, Python में while loop का उपयोग मुख्य रूप से dynamically changing condition के आधार पर loop में मौजूद statements को बार-बार execute करने के लिए किया जाता है।
Example: नीचे दिए गए example में, while loop एक infinite loop है, यानी यह तब तक चलता रहेगा जब तक break statement नहीं मिलेगा।
while True:
num = int(input("Enter a number to check if it is even or not, or enter 0 to exit the loop: "))
if num == 0:
print("Exiting the program. Goodbye!")
break
elif num % 2 == 0:
print(f"The number {num} is even.")
else:
print(f"The number {num} is odd.")
Output:
Enter a number to check if it is even or not, or enter 0 to exit the loop: 4
The number 4 is even.
Enter a number to check if it is even or not, or enter 0 to exit the loop: 7
The number 7 is odd.
Enter a number to check if it is even or not, or enter 0 to exit the loop: 0
Exiting the program. Goodbye!
Break Statement with Nested Loop:
Nested loop में, break statement directly जिस loop के अन्दर होगा program का flow break statement से सिर्फ उसी loop में बाहर (exit) होगा।
Example:
# Outer loop
for i in range(1, 4):
# Inner loop
for j in range(1, 4):
# Inner loop ko break karega jab j 3 ho
if j == 3:
break
print(f"i = {i}, j = {j}")
Output:
i = 1, j = 1
i = 1, j = 2
i = 2, j = 1
i = 2, j = 2
i = 3, j = 1
i = 3, j = 2
Continue Statement in Python
कभी-कभी हमें loop के अंदर किसी specific condition के true होने पर, loop के अंदर के कुछ statements को बिना execute किए यानी skip करके, loop को अगले iteration पर continue करना होता है। इसके लिए हम continue statement का उपयोग करते हैं।
Loop में जैसे ही continue statement मिलता है, उसके बाद loop में मौजूद बाकी statements को skip कर दिया जाता है और प्रोग्राम का flow फिर से loop के अगले iteration या condition पर चला जाता है।
Continue Statement Syntax:
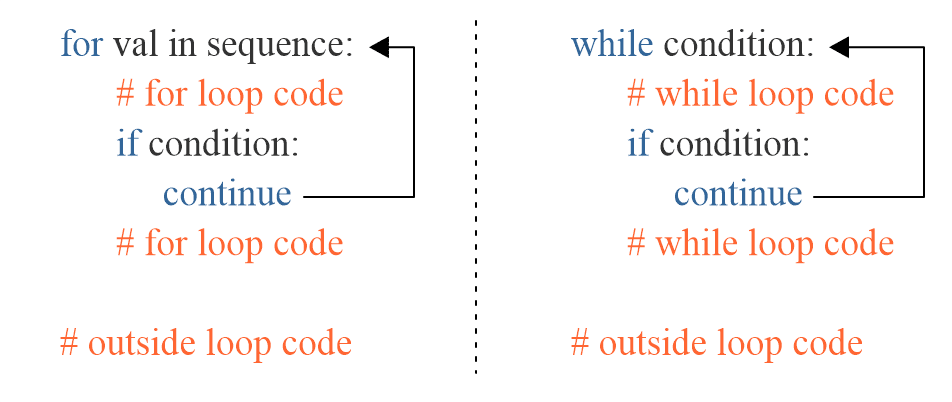
Continue Statement with For Loop:
नीचे दिए गए example में, जैसे ही list का कोई element number 5 से divisible होगा, continue statement की वजह से print statement skip हो जाएगा, यानी output में सिर्फ वो elements print होंगे जो 5 से divisible नहीं होंगे।
Example:
mylist = [24, 15, 28, 45, 78, 98]
for ele in mylist:
if ele % 5 == 0:
continue
print('Element:', ele)
Output:
Element: 24
Element: 28
Element: 78
Element: 98
Continue Statement with While Loop:
नीचे दिए गए example में, 1 से लेकर 10 तक सिर्फ odd numbers ही print होंगे। ऐसा इसलिए होगा क्योंकि जैसे ही variable num की value even number होगी, continue statement की वजह से print statement skip हो जाएगा और प्रोग्राम का flow फिर से while condition पर move कर जाएगा।
Example:
num = 1
# Loop from 1 to 10
while num <= 10:
# Agar number even hai
if num % 2 == 0:
num = num + 1
# Even number ko skip karna
continue
# Odd number ko print karna
print(num)
num = num + 1
Output:
1
3
5
7
9
Continue Statement with Nested Loop:
Nested loop में, continue statement केवल उसी loop के statements को skip करेगा, जिसमें वह directly मौजूद है।
जैसे, नीचे दिए गए program में, जब-जब variable j की value equal to 2 होगी, तब inner loop में मौजूद continue statement की वजह से print statement execute नहीं होगा।
Example:
# Outer loop
for i in range(1, 4):
# Inner loop
for j in range(1, 4):
# Inner loop ko continue karega jab j 2 ho
if j == 2:
continue
print(f"i = {i}, j = {j}")
Output:
i = 1, j = 1
i = 1, j = 3
i = 2, j = 1
i = 2, j = 3
i = 3, j = 1
i = 3, j = 3
Pass Statement in Python
बहुत बार programs बनाते समय ऐसा होता है कि हमें यह तो पता होता है कि if के साथ कौन सी condition लिखनी है, loop को कैसे और कब तक चलाना है, या function का prototype क्या होगा। लेकिन, हमने यह decide नहीं किया होता कि इनके अंदर कौन से statements लिखने हैं, यानी इनके block (body) को कैसे implement करना है।
ऐसे में, यदि आप if, loop, function, या class के block को खाली छोड़ते हैं, तो Python में आपको SyntaxError दिखाई देगा। इस SyntaxError से बचने के लिए और इनके block (body) को भविष्य में implement करने के लिए आप pass statement का उपयोग कर सकते हैं।
Python में pass statement का उपयोग if, loop, function, या class के empty block में placeholder या “do nothing” command के रूप में किया जाता है। यह syntax errors को रोकने और बिना implementation के सिर्फ code structure तैयार करने में बहुत उपयोगी होता है।
आइए अब देखें कि कैसे pass statement को if, loop, function, या class के empty block में placeholder के रूप में उपयोग किया जाता है।
1. If Statement में pass
जब किसी if या else block में कुछ भी execute नहीं करना हो (अभी के लिए), तो हम pass का उपयोग कर सकते हैं:
x = 10
if x > 5:
pass # Placeholder, भविष्य में यहाँ logic आएगा
else:
print("x is less than or equal to 5")
2. Loop में pass
अगर आप एक loop का structure बनाना चाहते हैं, लेकिन उस पर कोई operation नहीं करना चाहते, तो pass का उपयोग करें:
for i in range(5):
pass # Loop का logic बाद में जोड़ा जाएगा
while True:
pass # Infinite loop placeholder, आगे condition जोड़ेंगे
3. Function में pass
जब हम सिर्फ function का structure बनाना चाहते हैं और उसे बाद में implement करना चाहते हैं:
def mera_function():
pass # Placeholder function, future में logic implement होगा
# Function call
mera_function()
4. Class में pass
अगर आप एक class का structure बनाना चाहते हैं, लेकिन अभी उसमें कोई properties या methods नहीं जोड़ना चाहते:
class MeraClass:
pass # Placeholder class, बाद में properties और methods जोड़ेंगे
# Class का object बनाना
obj = MeraClass()
ऊपर दिए गए सभी example programs का कोई output नहीं आएगा और न ही कोई syntax error show होगा।
What’s Next: इस tutorial में हमने Python में important jumping control statements — break, continue, और pass को use करना सिखा। अगले tutorial में हम Python में List का उपयोग करना सीखेंगे।